You've reached the internet home of Chris Sells, who has a long history as a contributing member of the Windows developer community. He enjoys long walks on the beach and various computer technologies.
Tuesday, Apr 20, 2010, 4:27 PM in Oslo Featured Content
SQL Server Modeling CTP (November 2009 Release 3) for Visual Studio 2010 RTM Now Available
Here's what Kraig has to say about the November 2010 SQL Server Model CTP that matches the RTM of Visual Studio 2010:
A update of the SQL Server Modeling CTP (November 2009) that's compatible with the official (RTM) release of Visual Studio 2010 is now available on the Microsoft Download Center. This release is strictly an updated version of the original November 2009 CTP release to support the final release of Visual Studio 2010 and .NET Framework 4.
We highly recommend you uninstall and install in the following order.
- Uninstall any existing SQL Server Modeling CTP from Add and Remove Programs
- Uninstall Visual Studio 2010 and .NET Framework 4 Beta 2 or RC from Add and Remove Programs
- Install Visual Studio 2010 and .NET Framework 4
- Install the SQL Server Modeling November 2009 CTP Release 3.
If you are unable to uninstall SQL Server Modeling CTP from Add and Remove Programs for any reason, you can remove each component using the following command lines. You need to run all three in order to completely remove SQL Server Modeling CTP so you can install the new CTP:
M Tools: Msiexec /x {B7EE8AF2-3DCC-4AFE-8BD2-5A6CE9E85B3A}
Quadrant: Msiexec /x {61F3728B-1A7D-4dd8-88A5-001CBB9D2CFA}
Domains: Msiexec /x {11DA75C8-10AB-4288-A1BB-B3C2593524A7}
Note: These steps will not remove the SQL Server Modeling CTP entry in Add and Remove Programs but you will be able to install the new CTP.
Thank you and enjoy Visual Studio 2010!
Kraig Brockschmidt
Program Manager, Data Developer Center
Monday, Apr 5, 2010, 10:33 PM in .NET
The performance implications of IEnumerable vs. IQueryable
It all started innocently enough. I was implementing a "Older Posts/Newer Posts" feature for my new web site and was writing code like this:
IEnumerable<Post> FilterByCategory(IEnumerable<Post> posts, string category) {
if( !string.IsNullOrEmpty(category) ) {
return posts.Where(p => p.Category.Contains(category));
}
}
...
var posts = FilterByCategory(db.Posts, category);
int count = posts.Count();
...
The "db" was an EF object context object, but it could just as easily been a LINQ to SQL context. Once I ran this code, it failed at run-time with a null reference exception on Category. "That's strange," I thought. "Some of my categories are null, but I expect the 'like' operation in SQL to which Contains maps to skip the null values." That should've been my first clue.
Clue #2 was when I added the null check into my Where expression and found that their were far fewer results than I expected. Some experimentation revealed that the case of the category string mattered. "Hm. That's really strange," I thought. "By default, the 'like' operation doesn't care about case." Second clue unnoticed.
My 3rd and final clue was that even though my site was only showing a fraction of the values I knew where in the database, it had slowed to a crawl. By now, those of you experienced with LINQ to Entities/SQL are hollering from the audience: "Don't go into the woods alone! IEnumerable kills all the benefits of IQueryable!"
See, what I'd done was unwittingly switched from LINQ to Entities, which takes my C# expressions and translates them into SQL, and was now running LINQ to Objects, which executes my expressions directly.
"But that can't be," I thought, getting hot under the collar (I was wearing a dress shirt that day -- the girlfriend likes me to look dapper!). "To move from LINQ to Entities/SQL to LINQ to Objects, I thought I had to be explicit and use a method like ToList() or ToArray()." Au contraire mon fraire (the girlfriend also really likes France).
Here's what I expected to be happening. If I have an expression like "db.Posts" and I execute that expression by doing a foreach, I expect the SQL produced by LINQ to Entities/SQL to look like this:
select * from Posts
If I add a Where clause, I expect the SQL to be modified:
select * from Posts where Category like '%whatever%'
Further, if I do a Count on the whole thing, e.g.
db.Posts.Where(p => p.Contains(category)).Count()
I expect that to turn into the following SQL:
select count(*) from Posts where Category like '%whatever%'
And that's all true if I keep things to just "var" but I wasn't -- I was being clever and building functions to build up my queries. And because I couldn't use "var" as a function parameter, I had to pick a type. I picked the wrong one: IEnumerable.
The problem with IEnumerable is that it doesn't have enough information to support the building up of queries. Let's take a look at the extension method of Count over an IEnumerable:
public static int Count<TSource>(this IEnumerable<TSource> source) {
...
int num = 0;
using (IEnumerator<TSource> enumerator = source.GetEnumerator()) {
while (enumerator.MoveNext()) { num++; }
}
return num;
}
See? It's not composing the source IEnumerable over which it's operating -- it's executing the enumerator and counting the results. Further, since our example IEnumerator was a Where statement, which was in turn a accessing the list of Posts from the database, the effect was filtering in the Where over objects constituted from the following SQL:
select * from Posts
How did I see that? Well, I tried hooking up the supremely useful SQL Profiler to my ISP's database that was holding the data, but I didn't have permission. Luckily, the SQL tab in LinqPad will show me what SQL is being executed and it showed me just that (or rather, the slightly more verbose and more correct SQL that LINQ to Entities generates in these circumstances).
Now, I had a problem. I didn't want to pass around IEnumerable, because clearly that's slowing things down. A lot. On the other hand, I don't want to use ObjectSet<Post> because it doesn't compose, i.e. Where doesn't return that. What is the right interface to use to compose separate expressions into a single SQL statement? As you've probably guessed by now from the title of this post, the answer is: IQueryable.
Unlike IEnumerable, IQueryable exposes the underlying expression so that it can be composed by the caller. In fact, if you look at the IQueryable implementation of the Count extension method, you'll see something very different:
public static int Count<TSource>(this IQueryable<TSource> source) {
...
return source.Provider.Execute<int>(
Expression.Call(null,
((MethodInfo) MethodBase.GetCurrentMethod()).
MakeGenericMethod(
new Type[] { typeof(TSource) }),
new Expression[] { source.Expression }));
}
This code isn't exactly intuitive, but what's happening is that we're forming an expression which is composed of whatever expression is exposed by the IQueryable we're operating over and the Count method, which we're then implementing. To get this code path to execute for our example, we simply have to replace the use of IEnumerable with IQueryable:
IQueryable<Post> FilterByCategory(IQueryable<Post> posts, string category) {
if( !string.IsNullOrEmpty(category) ) {
return posts.Where(p => p.Category.Contains(category));
}
}
...
var posts = FilterByCategory(db.Posts, category);
int count = posts.Count();
...
Notice that none of the actual code changes. However, this new code runs much faster and with the case- and null-insensitivity built into the 'like' operator in SQL instead of semantics of the Contains method in LINQ to Objects.
The way it works is that we stack one IQueryable implementation onto another, in our case Count works on the Where which works on the ObjectSet returned from the Posts property on the object context (ObjectSet itself is an IQueryable). Because each outer IQueryable is reaching into the expression exposed by the inner IQueryable, it's only the outermost one -- Count in our example -- that causes the execution (foreach would also do it, as would ToList() or ToArray()).
Using IEnumerable, I was pulling back the ~3000 posts from my blog, then filtering them on the client-side and then doing a count of that.With IQueryable, I execute the complete query on the server-side:
select count(*) from Posts where Category like '%whatever%'
And, as our felon friend Ms. Stewart would say: "that's a good thing."
Friday, Apr 2, 2010, 10:37 AM in The Spout
College info for my sophomore
I went to a college planning sessions at my sons' high school not because I'm hung up on getting my Sophomore into a top school, but because I thought I'd get a jump on things. I learned I was actually behind.
For one, I learned that the high school has an online system that will do some amazing things:
- It will give my son a personality test and an interest test.
- From those tests, it will tell him what kinds of careers he might want to consider.
- Based on those careers, what major should he have.
- From the major, what schools around the country offer it.
- In those schools, what the entrance requirements are.
That means that my son can answer questions about personality and interests and draw a straight line through to what he needs to do to get into a school so he can learn to do the jobs he'll like and be good at. Holy cow. We didn't have anything like that when I was a kid.
Further, the online system has two complete SAT and ACT tests in it, so, along with the PSAT that he's already taking, he can do a practice ACT, figure out which test he's best at (my 34 ACT score was way better than my 1240 SATs) and just take that test, since most schools these days take both SAT or ACT results.
This is all freely provided by the high school and, in fact, they have counseling sessions with the students at each grade level for them to get the most from this system.
It's no wonder that 93% of students from this high school go on to 4 or 2-year college degree programs.
That was the good part.
The scary part is that my eldest, half way through his Sophomore year, is essentially half-way through his high school career. Colleges only see their grades through the end of Junior year, since most college applications are due in the middle of January of their Senior year at the latest. I have to sit down with my son and have the conversation about how "even if you get a 4.0 from now on, the best grades you can have are..."
Is it just me or is the world moving faster with each passing day?
Saturday, Mar 27, 2010, 1:53 PM in Tools
Updated the CsvFileTester for Jet 4.0
I was playing around building a tool to let me edit a database table in Excel, so I updated my CvsFileTester project to work in a modern world, including the 32-bit only Jet 4.0 driver you've probably go lying around on your HD.
Enjoy.
Sunday, Mar 21, 2010, 1:40 PM in The Spout
you may experience some technical difficulties
I've been futzing with the site and I've got more to do, so unexpected things may happen. Last weekend I screwed with the RSS generator and that caused a bunch of folks to see RSS entries again. This weekend I'm moving more of my static content into the database, so you may see a bunch of old stuff pop up.
Feel free to drop me a line if you see anything you think needs fixing. Thanks for your patience.
Thursday, Mar 18, 2010, 7:59 AM in The Spout
On Building a Data-Driven E-Commerce Site
The following is a preprint of an article for the NDC Magazine to be published in Apri.
It had been a long, hard week at work. I had my feet up when a friend called and popped the question: “Do you know how to build web sites?”
That was about a month ago and, after swearing to her that I spent my days helping other people build their web sites, so I should oughta know a thing or two about how to build one for her. After some very gentle requirements gathering (you don’t want a bad customer experience with a friend!), I set about designing and building bestcakebites.com, a real-world e-commerce site.
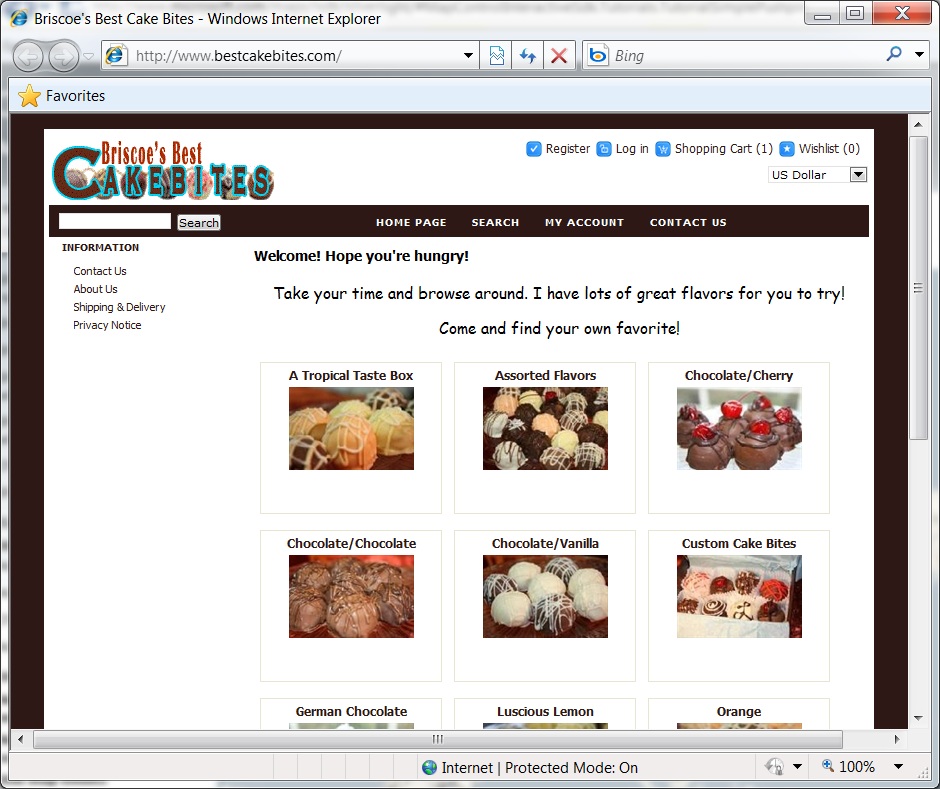
She didn’t need a ton of features, just some standard stuff:
· Built on a technology I already knew so I could have the control I needed.
· Listing a dozen or so products with pictures and descriptions.
· A shopping cart along with, ideally, an account system so folks could check their order status or reorder easily.
· Shipping, handling and tax calculation.
· Taking payment.
· Sending email notifications of successful orders to both the customer and the proprietor.
As it turns out, there are a bunch of ways to skin this particular cat, but because I was a busy fellow with a more-than-full-time job and a book I’m supposed to be writing, instead of falling prey to my engineering instinct to write my own website from scratch, I decided to see what was out there.
As it turns out, there’s quite a few e-commerce web site solutions in the world, several of them recommended by PayPal, as well as one that PayPal itself provides, if you don’t mind sending shoppers to their web site. And if fact, I did. Requirement #1 was that I needed complete control over the code and the look and feel of the site. I didn’t want to configure somebody else’s web site and risk going off of her chosen domain name or not being able to tweak that one little thing that meant the difference between #succeed and #fail. (Friend customers are so picky!)
The e-commerce solution I picked was the one I found on http://asp.net (I am a Microsoft employee after all): nopCommerce. It’s an open source solution based on ASP.NET and CSS, which meant that I had complete control when it wasn’t perfect (control I used a few times). It was far more than full-featured enough, including not only a product database, a shopping cart, shipping calculation and payment support, but also categories and manufacturers, blogs, news and forums, which I turned off to keep the web site simple (and to keep the administration cost low). Unexpected features that we ended up liking included product variants (lemon cake bites in sets of 8, 16 and 24 made up three variants, each with their own price, but sharing a description), product reviews, ratings and site-wide search.
The real beauty of nopCommerce, and the thing that has been the biggest boon, was that the whole thing is data-driven from SQL Server. To get started, I ran the template web site that was provided, it detected that it had no database from which to work and created and configured the initial database for me, complete with sample data. Further, not only was it all data-driven based on the products, orders and customers the way you’d expect, but also on the settings for the web site behavior itself.
For example, to get shipping working, I chose from a number of built-in shipping mechanisms, e.g. free, flat rate, UPS, UPSP, FedEx, etc., and plugged in my shipper information (like the user name and password from my free usps.com shipping calculation web service account)
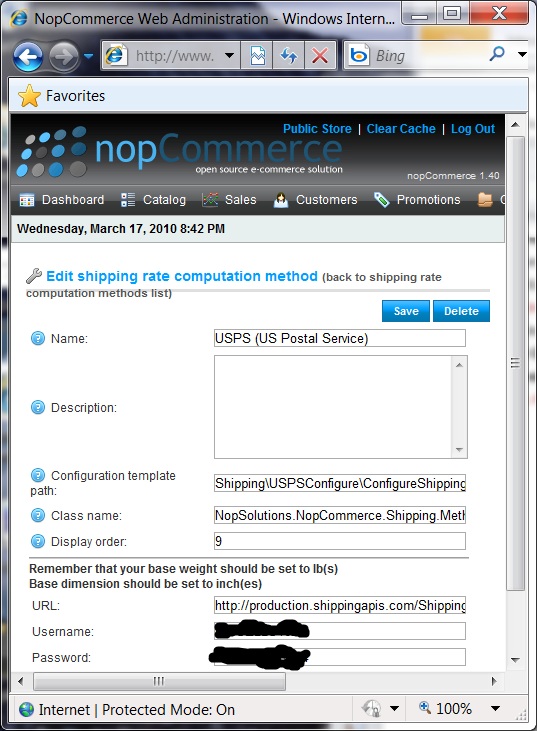
With this configuration in place, the next order the site took, it used that shipper, pulling in the shipping information from the set of size and weight measurements on the ordered products (from the database), calling the web service as it was configured (also from the database) to pull in the set of shipping options from that shipper, e.g. Express Mail, Priority Mail, etc., augmenting the shipping prices with the per product handling changes, and letting the user pick the one they wanted. All I had to do was use the administration console, tag each product with size information and tell nopCommerce that I’d like USPS today, please.
Everything worked this way, including tax calculation, payment options (we chose PayPal Direct and Express so that folks with a credit card never left our site whereas folks with PayPal logged into their account on the familiar paypal.com), localization, whether to enable blogs, news, forums, etc. Most of the time when I wanted to make a change, it was just a matter of flipping the right switch in the database and not touching the code at all.
As one extreme example of where the data-driven nature really came through was on the order numbers generated by the site. During testing, I noticed that our order numbers were monotonically increasing from 1. Having ordered from a competitor’s site, their order number was only 103, clearly showing off what amateurs they were (and the order itself took a month to arrive after two pestering emails, so it was clear how amateur they really were). I didn’t want us to appear like newbies in our order-confirmation emails (which nopCommerce also generated for us), so I found the Nop_Order table, and used SQL to increase the identity column seed, which it was clear was the origin of the order number:
DBCC CHECKIDENT (Nop_Order, RESEED, 10047)
From then on, every time an order came through, we protected experience simply because of the order number, which I changed without touching a line of code. If helping you “fake it ‘til you make it” isn’t enough reason to love a data-driven solution, I don’t know what is!
Thursday, Mar 11, 2010, 11:21 AM in The Spout
The incomplete list of impolite WP7 dev requests
In my previous list of WP7 user requests, I piled all of my user hopes and dreams for my new WP7 phone (delivery date: who the hell knows) onto the universe as a way to make good things happen. And all that’s fine, but I’m not just a user; like most of my readers, I’m also a developer and have a need to control my phone with code. I have a long list of applications I want to write and an even longer list of applications I want other developers to write for me.
Today at 1:30p is the first public presentations of how to do WP7 programming, so to affect the future, I have to get my feature requests for the Windows Phone 7 Series development environment posted now!
· I want the legendary Microsoft tools for code editing, UI design, debugging, deployment, version control, add-ins, project management, etc. Please just let me install the “Windows Phone 7 Series SDK” and have Dev10 light up with new project and project item templates et al.
· I definitely want to be able to write C#. Since Charlie’s mentioned Silverlight, it seems like I’ll be able to do just that.
· I want to be able to mark a program as useful for background processing, e.g. I’m writing Pandora, and let that trigger a question for the user as to whether to allow it or not, ideally with a message like “Pandora would like to continue to run in the background using XX% of your battery. Is that OK?”
· For most apps that would like to appear as if they’re running in the background, I want to register a bit of code to run when its own cloud-based notifications comes in, e.g. an new IM or sports score.
· I want to be able to access data from built-in apps, e.g. contacts, appointments, etc.
· Obvious things:
o Notification when the user switches orientation
o Access to the compass, GPS, network, camera, mic, etc.
o Access to the network using TCP, HTTP, Atom, AtomPub and OData.
o Low and high-level access to gestures
o A nice set of build-in standard controls, including simple things like text box and more complicated things like map and route
o Integration with existing apps, e.g. the ability to launch the map app from my own app at a specific location or to a specific route.
o Ability to create custom controls/do custom drawing.
o Serialization so I can keep user data between sessions. Notifications when my app is being whacked.
o App-selected keyboards for specific tasks, e.g. entering a URL.
That doesn’t seem like a very big list. I must be missing something. : )
Tuesday, Mar 9, 2010, 1:47 PM in The Spout
Creating a Lazy Sequence of Directory Descendants in C#
My dear friend Craig Andera posted an implementation of a function that descends into a directory in a "lazy" manner, i.e. you get the first descendant back right away and not after all descendants have been calculated. His implementation was in Clojure, a Lisp variant that runs on the Java VM:
(import [java.io File])
(defn dir-descendants [dir]
(let [children (.listFiles (File. dir))]
(lazy-cat
(map (memfn getPath) (filter (memfn isFile) children))
(mapcat dir-descendants
(map (memfn getPath) (filter (memfn isDirectory) children))))))
Craig was happy with this function because, even though it was a "mind-bender to write," it's easy to read and because C# "would almost certainly be at least somewhat more verbose."
Taking this as a challenge, I rewrote this program in C#, maintaining the laziness:
using System.IO;
using System.Collections.Generic;
namespace ConsoleApplication1 {
class Program {
static IEnumerable<string> GetDirectoryDecendants(string path) {
foreach (var file in Directory.GetFiles(path)) { yield return file; }
foreach (var directory in Directory.GetDirectories(path)) {
foreach (var file in GetDirectoryDecendants(directory)) { yield return file; }
}
}
}
}
The key hit here is the use of "yield return" which lets me return elements of an IEnumerable as I calculate them. This took me 5 minutes to write, my mind is straight and I find it fairly easy to read. I'll let you decide on the relative verbosity of each implementation.
Tuesday, Mar 9, 2010, 11:25 AM in The Spout
The incomplete list of impolite WP7 user feature requests
When I first moved from the combination of a dumb phone and a separate music player, I had modest requirements: phone calls, MP3 playback, calendar notifications, contact management, email, camera and solitaire. Even asking for only these seven things, my first smart phone was as life changing as my first laptop. I could do a great deal of my work while out and about, allowing me to have a much more productive work/personal life balance.
When I was first married, the word “love” didn’t seem big enough for what I felt for my bride. These days, the word “phone” doesn’t seem nearly big enough for the pocket-sized mobile device that I’m never without, like my wallet and my keys. Further, I expect my phone to replace my wallet and keys any day now, along with the Walkman, DVD player, TV, radio, book shelf, notepads, calculator, compass, alarm clock, wall calendar, newspaper, encyclopedia, dictionary, GameBoy, carpenter’s level, laptop, navigation device and landline it’s already replaced.
Now that I’ve been through several smart phones, including my favorites, the T-Mobile Dash and the iPhone 3G, I have a much longer, incomplete list of what I want from my Windows Phone 7 Series (and I know it’s incomplete because after I post this list, someone is going to remind me what vital things I missed : ).
· A calculator. It’s surprising how useful this is, including the scientific features.
· A battery that lasts at least 24 hours while I’m using Bluetooth, 3G, Wi-Fi, GPS, music and my apps. Oh, and please let me charge the thing with a standard connector (USB!) and let me use my phone while it’s recharging.
· An easy, high-quality way to run the music through my car stereo. The Sells Brothers and I like to jam!
· An easy way to switch back and forth to airplane mode. Or even better, can you make it so the device isn’t an FAA threat during takeoff and landing so I can stop reading the stupid magazine in the seat pocket in front of me for 5 minutes at the beginning and ending of my flights?
· Great auto-correct on my hard or soft keyboard entry. This is really the only way that allows my big fingers and the lack of tactile feedback to even work with a phone keyboard.
· Copy-paste: I can’t live w/o it anymore. Also, please include pasting into my phone during calls so I can stop memorizing 9-digit conference call IDs.
· I’d really love intelligent integration of music, i.e. keep it playing when I switch apps and not just the built-in Zune player, but 3rd party music apps, too (aka Pandora). Also, let me pause, next, previous while my phone is locked or I’m in another app. Finally, make sure to stop music when I get a call and start it back up again when my call is over. I love that.
· Full (!) calendar support:
o Sync’ing with Exchange and not Exchange.
o Recognition of phone numbers and addresses in my calendar appointments with links to dial/get directions.
o Reply All to an appointment so that I can let folks know I’m running late.
o Snooze on my meeting reminders (I can’t tell you how many times I’ve forgotten my meeting after the one and only 15 minute reminder).
o Show my appointments on my home page instead of making me dig into some app.
· Please provide a responsive UI, even if I haven’t rebooted in a week. Ideally I’d never have to reboot at all.
· Wireless sync’ing to my PC. My house is bathed in Wi-Fi; why make me connect a wire?
· Tethering so I can use my phone as a network connection for my PC. I’m paying for unlimited data – let me use it! And ideally make that wireless, too.
· Turn-by-turn directions! This won’t be ready until I go off course and I hear “recalculating” from my phone piped through my car stereo with Pandora playing in the background.
· There definitely needs to be an "app store" for phone apps, but also there needs to be a way to install apps from other sources without hacking my phone. Also, please let me install them on my SD card so I can take advantage of the extra memory.
· Let me install extra memory!
· Let me replace the battery! Batteries go bad over time and they need to be replaced on the go.
· I need a great audio book listening experience (bookmarks!) and a great ebook reading experience (formats!).
· I’d like some phone-wide search, including the ability to see where the result came from. I never want the email-only Contact from the list of everyone that I’ve ever received an email from – I want the real contact info that I’ve got cached on my phone.
· Full contact lookup, both personal and corporate (Exchange).
· Good camera (and flash): the one on my phone is the only one I ever use, as it’s the one I always have with me.
· Bluetooth and voice dialing for hands free operation (required by state law in both Washington and Oregon, where I spend most of my time). Also, I’d love the same integration with my Jawbone that I have with my iPhone, i.e. volume control and battery indicator.
· Apps I can’t live without:
o Evernote: I’m willing to move my data into OneNote so long as I can sync between the web, my phone and my PC.
o Social networking clients: IM, Twitter, an RSS/ATOM Feed Reader, YouTube and Facebook.
o Converter for currency, distance, volume, etc.
o A Compass.
o A Flashlight. I have used the ambient life from my phone to get myself out of the forest in pitch blackness. Without it, I’m sure I would’ve been hacked to pieces by Jason or Michael Meyers.
o TripIt, Movies, OpenTable, UrbanSpoon, Mint: I use these all the time.
o Shazam: Before this app, I used to record snippets of songs and email them to my son would be charge me $.50/ea. to find the title and artist so I could grab them for my phone. Shazam has cut out the middle man and represents ~100% of the music I purchase these days.
o Skype or some other good way to use my phone to do IP Telephony (or even IP Video Conferencing)
o Tetris! I guess there are other casual games in the world, but that’s mine.
Because the WP7 hasn’t shipped yet, I can pile all of my hopes and dreams on it and, like everyone else not on the WP7 team, I have very little idea of whether my hopes will be fulfilled, but that doesn’t stop me from dreaming. So now it’s your turn – what did I miss? : )
P.S. I know that lots of phones have a subset of these features and I’m sure someone will tell me that, with the correct config, I can make their favorite phone do all these things. I know that’s going to happen because whenever I complain about a missing feature in the Visual Studio editor, some emacs guy says, “Oh, you can do that with Alt+Shift+Left Elbow in my editor!” I don’t care about what your phone can do. No phone’s UI has stirred me like the WP7’s UI. That’s the one I want to use, so that’s the phone I’m going to bang on ‘til my incomplete list is complete.
Friday, Mar 5, 2010, 4:19 PM in Oslo Featured Content
"Deep Fried Bytes Podcast": Lars on SQL Server Modeling
Here's how the Deep Fried guys describe episode 45: "At PDC 2009, 'Oslo' was renamed to SQL Modeling and it left a lot of developers scratching their heads. What better way to sort it all out than to talk with someone deep into the stack. We sat down with Lars Corneliussen to see how this is all going to turn out and it what it means for developers. Definitely an interesting show as it paints a different picture about where things are going with 'M', 'M' Grammar, SQL modeling, Entity Framework, Quadrant and so on." Check it out!
Thursday, Mar 4, 2010, 10:13 AM in Oslo Featured Content
SQL Server Modeling CTP (November 2009 Release 2)
An update of the SQL Server Modeling CTP (November 2009) that's compatible with Visual Studio 2010 RC has been released on the Microsoft Download Center. This release is strictly an updated version of the original November 2009 CTP release to support Visual Studio 2010 and .NET Framework 4 RC. It contains no other fixes outside of those required to work with the new RC. Enjoy!
Wednesday, Mar 3, 2010, 5:21 PM in The Spout
Please don't run apps in the background on my WP7 phone!
When I was but a wee lad, I learned that when it came to my computer, I was often going to be waiting on something, whether it was the CPU, the IO or the memory. Now that I'm all grown up and spending a great deal of time on handheld mobile devices, I've discovered a whole new thing I'm waiting on: charging the battery.
In the bad old days of DOS, I spent a disturbing amount of time working on my autoexec.bat and config.sys files to optimize the loading of drivers and TSRs (DOS programs that run in the background for you whippersnappers). Now, instead of optimizing for memory usage on my PC, I spend my time optimizing for power consumption on my phone, e.g. turning off 3G and Bluetooth when I don't need them, turning down the polling frequency on my SMTP mail accounts and spreading power adapters everywhere in my world where I sit for more than 5 minutes. The single most important feature on my phone is that's it's on and the way power is managed on my iPhone means that this is often not the case. Sometimes I pine for my Windows Mobile Dash for just that reason; it ran for days instead of hours.
And as bad as this power situation is, it would be even worse if my phone ran more than one app at a time. I don't worry about random apps from the AppStore using too much memory or crashing; I worry about them eating my battery and killing my iPhone in the middle of a route to somewhere I've never been. By not allowing background apps to run, Apple is trying to do the right thing but (although my battery life still sucks). I don’t have personal experience with Google phones, but since they do allow background apps to run, I have to imagine battery life is an even bigger problem.
So, when I see people lobbying for background apps on the new Windows Phone 7 Series, all I can say is, you don't want it. What you want is for work to go on in the background for you without the cost in power.
Oh, I want to listen to my MP3s or Pandora while I answer my email like everyone else, but I don't want every financial/IM/email/social/sports app I download sucking down my battery life because it feels itself to be more important than everything else on my phone. I want those apps to notify me when something I care about happens but I don't want the processing to discover such events to happen on my phone - I want the processing to happen in the cloud.
You may recall my piece about how important storage in the cloud is for moble devices. Let's let somebody else scale and manage the storage so we can leverage it. In the same way, we want to leverage CPU and power in the cloud, saving local resources for cool graphics, twitch games and streaming my "Pink" channel.
Of course, if we're going to push the processing to the cloud, I'm going to need an efficient and easy way to write my WP7 apps to be notified so I can do the actual processing that needs to happen on the phone. And that all needs to happen while I'm navigating and playing my bad girls party mix.
I'm saving my WP7 phone battery for important things after all.
Monday, Feb 22, 2010, 11:31 PM in Oslo Featured Content
Update for SQL Server Modeling CTP and Dev10 RC
We are currently preparing a release of the SQL Server Modeling November 2009 CTP that will install and operate with Visual Studio Release Candidate. We expect to make this release available the first week of March and will make an announcement here at that time.
We are also planning for another release of the November CTP that matches the final Visual Studio product (RTM) when that product becomes generally available.
Monday, Feb 15, 2010, 2:23 PM
Windows Phone Series 7 Link Roundup
I was swamped this morning, so didn't get to see the Windows Phone Series 7 stuff live. When I did finally get to poke my head out, Scott Stanfield and Damir Tomicic had the list of links all set for me to follow. Thanks, guys!
- Steve Ballmer’s Keynote this morning in Barcelona, Spain
- Channel 9 Hands On
- Main site with good micro-demos
- Gizmodo
- Gizmodo hands-on
Now I just have to let my AT&T contract run out and find a buyer for my iPhone. Can't wait!
Tuesday, Feb 9, 2010, 5:55 PM in Tools
Entity Designer Database Generation Power Pack
If you like Model-First design in Entity Framework, you're going to love the Entity Designer Database Generation Power Pack. The original Database Generation feature in the Entity Designer in VS 2010 is extensible via Windows Workflows and T4 Templates. This Power Pack builds on these extensibility mechanisms and introduces the following:
- Basic Table-per-Hierarchy support. This is represented by the “Generate T-SQL via T4 (TPH)” workflow.
- The SSDL and MSL generation pieces can now be tweaked through T4 templates, both in TPH and TPT strategies through the “Generate T-SQL via T4 (TPT)” and “Generate T-SQL via T4 (TPH)” workflows.
- Direct deployment and data/schema migration are available through the “Generate Migration T-SQL and Deploy” workflow. This workflow will use the Team System Data APIs to diff our default T-SQL script against the target database and create a new script which will perform non-invasive ALTERs and data migration where necessary.
- A new user interface will now display when “Generate Database from Model” is selected – this acts as a “workflow manager” which will present to you our default workflows and allow you to create your own, customizable workflows based on your own strategy, script generation, and deployment requirements.
Highly recommended. Enjoy!