You've reached the internet home of Chris Sells, who has a long history as a contributing member of the Windows developer community. He enjoys long walks on the beach and various computer technologies.
Saturday, Nov 29, 2003, 12:00 AM in The Spout
The Wonder that is XAML's Extended Attribute Syntax
Saturday, November 29, 2003
I've been playing with GridPanel today and really like how elegant it is. If I say the following:
<GridPanel> <SimpleText>Testing</SimpleText> <SimpleText>1</SimpleText> <SimpleText>2</SimpleText> <SimpleText>3</SimpleText> </GridPanel>
I get the equivalent of an HTML table with four rows with each SimpleText element forming a cell like so (augmented with Border elements not shown above to accentuate the cells):
If I want to split the data into columns, I can do that using the Columns attribute:
<GridPanel Columns="2"> <SimpleText>Testing</SimpleText> <SimpleText>1</SimpleText> <SimpleText>2</SimpleText> <SimpleText>3</SimpleText> </GridPanel>
Which gives me two rows of two columns each:
That's all nice and simple, but still flexible. For example, if I want to set the Testing string to cover two columns, I can give it an ID in the XAML and call the GridPanel.SetColumnSpan static method in code, e.g. when the Window loads:
<!-- xaml --> <Window ... Loaded="window1_Loaded"> <GridPanel Columns="2"> <SimpleText ID="testingSimpleText">Testing</SimpleText> <SimpleText>1</SimpleText> <SimpleText>2</SimpleText> <SimpleText>3</SimpleText> </GridPanel> </Window>
// C# void window1_Loaded(...) { GridPanel.SetColumnSpan(testingSimpleText, 2); }
This code yields 3 rows arranged as follows:
This is all fine and makes you appreciate the elegance of the GridPanel. However, that's not what inspired me to write this entry.
Remember that the SimpleText element serves as a cell in the GridPanel. Even better, the SimpleText element doesn't know it's serving as a cell in a GridPanel. The architecture of Avalon is loosely-couple so that all of the elements don't need to have intimate knowledge of each other. This loose coupling is really great for future elements that some developer wants to host in a GridPanel or that want to host SimpleText elements. Still, I'd like to be able to set GridPanel-related properties for each "cell" on the cell element itself, even if the cell element doesn't know it's a child of a GridPanel. And that's what the extended attribute syntax in XAML lets me do. Instead of writing the code, I can just do this:
<GridPanel Columns="2"> <SimpleText GridPanel.ColumnSpan="2">Testing</SimpleText> <SimpleText>1</SimpleText> <SimpleText>2</SimpleText> <SimpleText>3</SimpleText> </GridPanel>
This dotted attribute syntax allows me to set a GridPanel setting for the SimpleText element w/o any knowledge from the GridPanel of the SimpleText or vice versa. That means that if I define the FooBar element tomorrow, I can set it's GridPanel.ColumnSpan on it when it's used inside a GridPanel w/o extending GridPanel. This also means that if FooBar has a Quux per-child property, I can set the FooBar.Quux attribute of the SimpleText element w/o extending SimpleText. Nice!
BTW, if you'd like to see the code that provides the GridPanel in "outline mode" as above, it looks like this:
<Border Background="black" Width="100%" Height="100%"> <GridPanel Columns="2"> <Border Background="white" GridPanel.ColumnSpan="2"> <SimpleText >Testing</SimpleText> </Border> <Border Background="white"> <SimpleText>1</SimpleText> </Border> <Border Background="white"> <SimpleText>2</SimpleText> </Border> <Border Background="white"> <SimpleText>3</SimpleText> </Border> </GridPanel> </Border>
Notice that the GridPanel.ColumnSpan is on the Border element, not the SimpleText element. That's because it's the Border that's the cell of the GridPanel, not the SimpleText.
Monday, Nov 24, 2003, 12:00 AM in The Spout
I don't know UIs, but I know what I like...
Monday, November 24, 2003
The user in me sees Longhorn and dreams of software without limits. The engineer in me sees Longhorn and is scared of software without limits. An engineer's days are defined by requirements and restrictions. Those restrictions come not only from the capabilities of the tools and technologies being bolted together, but also accepted practice. For years, I've been comfortable designing "rich" client applications because a) my tools and technologies haven't let me do much that was really "rich" and b) because "rich" was pre-defined by existing applications.
In fact, when I started programming Windows 3.1, I adopted a single 3-step UI design process:
- Figure out the the requirements of the particular UI doodad I needed
- Find my favorite example of it in Visual Studio, Word or Outlook
- Make User/MFC/WTL/WinForms do that
It doesn't take much to design an application that looks like this:
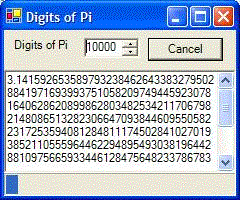
or even one that looks like this:
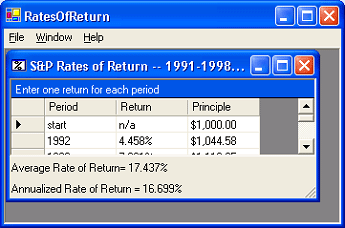
when the process is merely aping existing apps. I was even encouraged to use this kind of UI design by books with color pictures from well respected authors:
When the web came along, things were scary because all the guidelines were gone. However, at first the limitations were strict, so I didn't have any trouble building sites that looked like this:
but when a few years later, the limitations faded away and I was expected to conceive of and implement stuff like this:
I didn't have a clue. Luckily, the web "way" settled down over the years so that we figured out basically how to design web sites:
Plus, limitations took hold as we realized that you could build pretty much anything you wanted unless you wanted it to work on someone's machine besides yours, which turned the screws down pretty tightly.
Still, while the emerging conventions helped me figure out what I wanted my own web site to look like in general, I had to *pay* for help from a otherly-skilled professional before I could get the specifics:
This is where it got scary until I had the one application designed for which I would ever need a designer. With my web site out of the way, it was smooth sailing.
That is, until Longhorn and Avalon came along.
Avalon drops the bottom out of the limitations and the guidelines again, leaving guys like me in a lurch. Oh sure, I've done pretty much everything there is to do in this industry, from coder to President, from docs to QA, from project management to *shudder* marketing. However, what I haven't done and always hoped to avoid was design the graphics that go along with an application. Those were always things that I could hire done, most often as a deductible expense.
But now what do I do? MS doesn't provide MSDN authors a "graphic artist" budget. We do have very talented graphic artists at MSDN, but what's my boss going to say when they're swamped doing graphics for my pet projects and not the public-facing web site?
And standard bitmap graphic artifacts aren't enough; I need *vector* graphics. Even I can see that while this is fine:
nobody is expanding sol.exe's main window to get more "green space":
To solve problems like these, we need vector graphics that look good when they're scaled, which I understand is roughly 10x harder than bitmaps (which are already beyond me).
But just the graphics aren't enough, either. MS is busy redesigning the Windows "user experience" to include the best of Windows and the web:
Apps are supposed to behavior sensibly (which I can handle) *and* look cool (where do I learn to make *that* happen?!?).
In fact, taking the next step from the budding UX guidelines, we seem to be heading into a place where if I want to build my own sol to fix the scaling, I'll be trying to fit a "menu system" on the front like the gaming guys do:
and like so:
In fact, I've heard tell that there's a special guy that designs these pre-game antics separately from the rest of the game just so folks think the game is cool as soon as it starts, long before you're actually playing it. How can I stand up to that kind of pressure? I was pretty damn happy with myself when I made blocks fall and stack:
And while this is the only scalable game of its kind of which I know (I was ready for Avalon a while ago, apparently : ), it still has the standard un-cool "menu system" that Windows comes with out of the box.
And there's no relief in sight! Avalon, by taking advantage of 3D hardware and displays that haven't even been invented yet, along with intrinsic support for every kind of media and animation that I'm familiar with, blows right by the limitations we've grown to know and love in Windows and web applications. Now we're into the world of game programmers, where if you can imagine it, you can do it.
But where am I going to get the graphics for what I can imagine?
How do I imagine a user experience when MS is literally still writing the book on it and they haven't shipped a Longhorn Visual Studio, Word *or* Outlook?
Graphic/user experience designers of the world, please be gentle; the engineers are spooked and could stampede at any moment...
Know where I can get some graphics/user experience designers cheap?
Saturday, Nov 15, 2003, 1:49 PM in The Spout
My First Meeting with BillGs Technical Advisor
Here. The one where I meet BillG's Technical Advisor and am charmed into volunteering for more work.
Saturday, Nov 15, 2003, 12:00 AM in The Spout
My First Meeting with BillG's Technical Advisor
Saturday, November 15, 2003
In my continuing quest to take advantage of unique experiences at Microsoft for those that aren't able to, I spent an hour in a meeting with Bill Gates's Technical Advisor, Alexander Gounares, earlier this week. Among other things, Alex is responsible for getting answers when Bill asks questions like "Hey, what are we doing about adding air conditioning to Windows?"
I've had meetings with senior execs at MS before and I always end up saying stuff that upsets them. This time, the guy was so nice, I just wanted to nod my head and do whatever he asked of me. In fact, the thing I volunteered to work on (a "vision" whitepaper exploring a new application of technology that's still under development) wasn't so much that he asked me to do it, but rather because he asked for a volunteer and made eye contact with me in such a heart-warming way that I couldn't say no. (Of course, my boss kicking me under the table didn't hurt, either... : )
BTW, in case you wondered, while the Sr. VP I upset knew me from my blog, Alex didn't know me from Adam. : )
For folks keeping track, in my six month career at MS, I gotten to do *lots* of cool stuff:
- Launch the Longhorn Developer Center
- Hang out back stage at the PDC, including preparing to act as Chris Anderson's demo understudy and helping ship one of MSDN's new features (annotations)
- Write a Think Week paper for BillG and receive his comments back on it
- Meet Anders Hejlsberg, Eric Gunnerson and Robert Hess
- Have meetings with several execs, including one Group VP, one Sr. VP and BillG's TA
- Crash a WinFX API review
- Introduce MS to the joy of a DevCon
- Help get my PUM blogging
- Invent an extension to RSS that is getting fairly wide implementation, e.g. SharpReader, RssBandit and .Text.
- Appear on The .NET Show
- Get a few mentions in the press as an official MS employee (including one that my Mom saw that made her very proud : )
- Keep up contact with the community as much as I'm able via email, mailing lists, newsgroups, articles, conference talks and appearances
I list these not to brag, but to reflect on how many cool things there are to do at MS. I assume it's all down hill from here. : )
Monday, Nov 3, 2003, 10:34 PM in The Spout
Time For An Exciting Career In Electronics
Here. The one where blogs and RSS have removed the inefficiency in the market in which I make my living. Luckily, few people make full use of such technologies, so I may be able to make the mortgage for a few more months...
Monday, Nov 3, 2003, 9:34 PM in The Spout
In Search of Expertise
Here. The one where I finally figure out that membership in a profession does not imply expertise in that profession. For those of you saying "duh," that's not helpful... : )
Monday, Nov 3, 2003, 12:00 AM in The Spout
Time For An Exciting Career In Electronics
Monday, November 3, 2003
Depending on how you look at it, what I'm best at in the whole world has either become completely unnecessary or incredibly easy. The skill that I spent most of the last 10 years learning was to look at a group of types, APIs, reference docs, headers, source code, etc., distill it down to a set of architectural intentions and then to weave a story through the whole thing to make sense of it for developers that weren't able to glean the intensions themselves (most often because they had real jobs). I call this exhaustive search for intention officially at an end. I'm no longer needed except as a gatherer for those not yet facile in RSS aggregators (which, luckily for me, is still a large number : ).
Blogs like Chris's, Scott's and Raymond's go way beyond the "what" that docs and web sites and even mailing lists/newsgroups/web forums have typically stuck with and gone right into the "why." And not only that, but Chris is actually taking that next step on his blog. In the old days, before my favorite technology company became so damn open, if I wanted to comment on something, I had to painstakingly track down the right people at Microsoft, make friends with them (which first demanded that I learn social skills) and then convince them that someone outside of MS might actually have something intelligent and useful to say. This often involved years of stalking 'softies in mailing lists and conference halls and now Chris is letting anyone give him feedback to which he actually listens!
What use a hack like me that doesn't invent something, but merely figures out how existing things work so that I can tell someone about it if the guy that invents it does that part, too?!? I wonder if there are slots left in the vocational technical school near my house...
Monday, Nov 3, 2003, 12:00 AM in The Spout
In Search of Expertise
I had an interesting insight while watching Episode II: Attack of the Clones (I had a hankering to see Yoda kick some butt, OK?!?). The definition of expert is someone that just does whatever it is they do; they don't think about it. Everyone else is just learning. What drove this home for me was when Anakin heard of his mother being taken and how everyone else had failed or died looking for her, Anakin started out after her without any planning whatsoever. *That's* an expert. Of course, he had The Force and the rest of us have to live with an average amount of midichlorian, but he'd done what I've seen other experts do, too: unconscious competence.
The reason that this is such an insight for me is that for my entire life, I've always felt that there were special people in the world that just know what they're doing. These people worked in companies or were members of a profession and by their association with those organizations, I felt that they must be experts. I mean, how could someone be a member of professional for any length of time and not strive for mastery of the professional? It was watching Anakin that it finally sunk in that mere association is not enough; most organizations, no matter how high their standards, do not stop non-experts at the door. Experts are the ones inside the organizations that rise to the top, that stand out, that set examples for other members. Expertise is the thing that separates a moderately smart person studying up on a topic for a while from someone that really understands something down to their bones. This is what separates me catching up to my financial advisor after 6 months of study from a *real* money manager. That's what separates a new computer science graduate from me.
That's not to say that I couldn't be better than some "real" money managers one day or that the new college grad couldn't be better then me on day one. What it does say, however, is that encountering a true expert is a rare thing. Because of that rarity, I believe that most reasonably intelligent people can, with study and practice, get good at practically anything that they set their mind to. This means that I can, with a reasonable amount of study and practice, check the work of my financial advisor or my accountant or a plumbing contractor and come up with things that they don't. I know that this is true because I've done it (not with the plumbing though, I'm practically clueless there : ). The amount of study I needed to catch up with my professional service provider doesn't make me an expert, of course, because it's highly unlikely that either of them is an expert.
So, now that I've settled the question of whether membership in a profession makes one an expert (it doesn't), that leaves two questions:
- How do you recognize expertise? I know it when it occurs in something that I'm familiar with, e.g. computer goo, but what about in a discipline where I'm not versed?
- How do you obtain expertise? I've got this down to a science for programming APIs, but can these techniques be applied to other disciplines?
Comments on my continued quest for expertise welcome.
Monday, Nov 3, 2003, 12:00 AM in The Spout
My First PDC as a Microsoftie
After all the preparation, planning and sheer work, it's a letdown for the PDC to actually be over. It was an amazing experience for me to be "back stage" at a PDC, the only conference I'd spent my own money on for years (although as a speaker, they let me in gratis this year : ).
Things I Heard:
As a Microsoft employee, my job is to listen and take feedback home. Here's what I heard:
- Longhorn, Avalon/XAML, Indigo, WinFS, ClickOnce: good
- Improvements in VS.NET and ASP.NET: good
- Indigo and ClickOnce on existing versions of Windows: good
- Avalon not available on
existing versions of Windows: not so good
(Avalon changes things all the way to the device driver level, so retrofitting it to existing versions of Windows is impractical) - Not being able to download the
PDC Longhorn and VS.NET Whidbey bits: not so good
(MSDN subscribers can request the CDs to be sent to them) - Developers
that didn't go to the PDC and don't have MSDN subscriptions not getting PDC
bits: not so good
(the public beta in the summer of 2004 will open this up considerably) -
The Longhorn Developer Center features and content: good
(whew : ) - Having all kinds of Microsofties at the PDC and on the newsgroups and mailing lists to answer questions and take feedback: good
- Publicly contributed annotations on the Longhorn SDK content: good
- Longhorn bits running under Virtual PC: good
- Longhorn bits amazingly functional and stable: good
- Longhorn bits not especially
speedy: not so good
(these are the earliest OS bits we've ever given to folks -- Longhorn will have years to improve in this and every other regard) - Availability of PDC network, both wired and
wireless: not so good
(don't know why this happened) -
Stacking up outside the rooms of overflowing sessions: not so good
(although MS attendees were very good about giving up their seats to paying customers) - Microsoft's commitment to pushing managed code so far into the OS: good
- Lots of folks interested in contributing to
the Longhorn developer community: good
(if you're planning something, please let me know)
Things I Got To Do:
Also, as a first timer backstage at the PDC (and a long-time member of the Windows developer community), I got to do all kinds of fun things:
- Give a pre-conference tutorial on WinForms with Rocky Lhotka, a fellow giant Minnesotan with whom I'd never before had a chance to work
- Prepare Sunday night to do the keynote demo in Chris Anderson's place on Monday morning with Don Box and Jim Allchin in case Chris couldn't make it past the fires in time
- Hang out on the PDC keynote stage late at night, soaking up the Chairman of the Board and Sr. VPs doing their last minute preparations (and inadvertently making career-limiting statements : )
- Watch Don and Chris and Jim pull off an amazing all-code keynote that will go down in history as one of the PDC's best
- Hang out at the Ask The Experts session, coding up repros and solutions to WinForms questions alongside Mark "Mr. WinForms" Boulter
- Wandering the streets of downtown LA with Frank Redmond looking for Tim Ewald's surprise birthday party
- Answer questions instead of ask them at the MSDN booth
- Being interviewed by Jon Box of Sys-Con Radio and an old ATL student who blames me for his career choices
- Spent a lot of time telling people about MSDN Developer Centers, where my friends at MSDN work very hard to pull information out of the vast MSDN library and organize it for particular audiences, e.g. C#, VB.NET, ASP.NET, Security, VS.NET, the .NET Framework, Architecture, etc.
- Shake hands and sign autographs and have my picture taken with all kinds of old friends and ex-students and readers and conference attendees
- Gush like a fan-boy at Tim O'Reilly
- Watch a block full of cars all go backwards one night getting back into "first positions" (it was California, after all : )
- See the 4' high by 20' long WinFS schema and realize just how much work the WinFS guys are actually doing to capture "Everyday Information"
- Send out the official welcome to the WinFX newsgroups
- Make tweaks to several potential keynote demos at the last possible moment
- Do an interview with .NET Developer Journal that let me tell my side of all kinds of stories
- Get all kinds of feedback from internal and external folks saying nice things about the project that has consumed me for the last 6 months: the Longhorn Developer Center
- Go to a 1am show of The Metal Shop heavy metal "mock 'n' roll" band at the Viper Room on the strip in Hollywood with John Shewchuk and Matt Pietrek and screaming my voice raw to the lyrics
- Meet Sunny Day live and in person
- Hang out with Matt Pietrek, one of my personal heroes, along with Tim and Sarah and most of my closest friends (where was Craig?!?)
- Shake my tail feathers to yet another amazing Band on the Runtime gig
- Attend all kinds of technical sessions on the technologies of Longhorn so that I can continue to live the Longhorn life for those developers that aren't yet able to
- Start the collection of items for a Longhorn Developer FAQ which I'll post when it reaches critical mass
- Hang out with architects from two of the three main pillars of Longhorn (Don Box, Indigo and Chris Anderson, Avalon) at the The Kettle in Hermosa Beach
- Share a room with the 19-year old .NET wunderkind, Ryan Dawson
At this PDC, I definitely got to see how the sausage was made, but that still didn't take away from the deep, rich flavor. Recommend.
Thursday, Oct 23, 2003, 1:23 PM in The Spout
MSDN Wants You
Here. Want to write for MSDN online? Here's how.
Monday, Oct 20, 2003, 8:51 AM in The Spout
ASP Alliance reviews WinForms Programming in C#
Here. I don't know why an ASP site is reviewing a WinForms book, but they do a thorough job and, of course, I like their conclusion: "If you are looking for a Windows Forms book, don’t let this one slip out of your hands. The book has such insight and experience included that every reader, beginner and professional, will get benefit from reading it."
Monday, Oct 20, 2003, 12:44 AM in The Spout
Every Vain Author's Best Friend
Here. Rory built me an RSS feed to track book reviews on Amazon.com. And then he thanked me for letting him do it. You're welcome, Rory... BTW, to complete your Vain Author's Toolkit, you'll need junglescan.com to track a portfolio of books' sales rank. If those ain't web services, I don't know what are!
Wednesday, Oct 15, 2003, 9:14 AM in The Spout
How Does One Obtain Inside Information?
Here. The one where I list the steps I use to figure out stuff that isn't documented.
Wednesday, Oct 15, 2003, 12:00 AM in The Spout
How Does One Obtain "Inside" Information?
Wednesday, October 15, 2003
I got an email today from John Reilly today asking me a question I get from time to time:
I got my signed copy of your book. It is a great read. You have information that a friend and I have been trying to dig out for weeks.
Which leads me to our question. How do you find out all those details? They are not to be found in the MSDN documentation, as far as we can tell. Do you just experiment, like we do? We have been unable to find any .NET training that is much more than how to use the IDE. Alan and I crave detail, meat, the stuff of your book. Seriously, how does one obtain this seemingly inside information? We are personally and professionally motivated weenies.
Now that I'm a Microsoft employee, I have access to not only the source for most of what I'm researching, but the architects and internal mailing lists populated by the developers on the project, so that really is "inside information." However, over the 8+ years that I was a contributing member of the Windows developer community, 99% of what I've done has not been based on inside information. So, before I had access to internal info, how did I figure stuff out if the docs don't answer my questions? I do the following, in order of decreasing frequency:
- I experiment, writing hundreds of projects consisting of 10 lines of code or less to experiment with particular features
- I read the source, even if that means reverse engineering it first (Reflector is fabulous for .NET code)
- I search on the Microsoft-specific Google search page
- I IM, email and call my friends who know more than I do (which is most of them : )
- I ask questions on my favorite mailing lists and newsgroups
- As a last resort, I ask my friends at MS. Buying a Microsoftie a beer at a conference, being smart in online forums and saying nice things about their technologies are all excellent ways to gain friends at MS
Friday, Oct 10, 2003, 11:08 AM in The Spout
Amazon giving 30% off WinForms Programming in C#
Here. I think those Amazon guys knew I was talking about them...